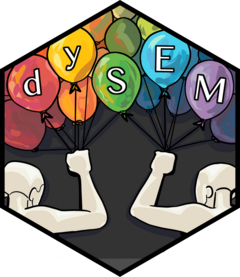
A Function That Fits and Compares Competing Dyadic Uni-construct Models
Source:R/outputUniConstructComp.R
outputUniConstructComp.Rd
This function takes the outputted object from scrapeVarCross()
along with the corresponding dataset and automatically tests competing
uni-construct dyadic models for the latent variable under consideration.
It inspects four possible model variants:
Bifactor (scripted via
dySEM::scriptBifac
)Hierarchical (scripted via
dySEM::scriptHier
)Correlated Factors (scripted via
dySEM::scriptCor
)Unidimensional (scripted via
dySEM::scriptUni
)
Usage
outputUniConstructComp(
dvn,
dat,
indexes = c("df", "chisq", "cfi", "rmsea", "bic", "GenTEFI"),
...,
gtTab = FALSE,
writeTo = NULL,
fileName = NULL
)
Arguments
- dvn
Input dvn list from
scrapeVarCross()
.- dat
Input data frame containing the dataset for model estimation.
- indexes
Input character vector specifying which index(es) to return. Default is
c("df", "chisq", "cfi", "rmsea", "bic", "GenTEFI")
. Note:Valid entries include "GenTEFI"—the Generalized Total Entropy Fit Index (see Golino et al., 2024)—and those from
lavaan::fitMeasures()
.If "chisq" is entered, chi-squared difference tests are automatically performed via
lavaan::lavTestLRT()
, and the resulting p-values are added to the output.
- ...
Additional arguments to be passed to
lavaan::cfa()
, allowing users to customize model estimation settings. By default, the models are fit with maximum-likelihood estimation (estimator = "ml"
) and missing data are handled via listwise deletion (missing = "listwise"
), as perlavaan::cfa()
's default behaviour.- gtTab
A logical input indicating whether to generate the requested index(es) for each fitted model (requested via the
indexes
argument) ingt::gt()
table object format (TRUE
). Users can also apply thewriteTo
argument if they wish to export thegt::gt()
table object.- writeTo
A character vector string specifying a directory path to where the
gt::gt()
table object should be saved. If set to ".", the file will be written to the current working directory. The default isNULL
, and examples use a temporary directory created bytempdir()
.writeTo
is only relevant ifgtTab = TRUE
.- fileName
A character string specifying a desired base name for the output
gt::gt()
file. The resulting base name will automatically be appended with a.rtf
file extension.fileName
is only relevant ifgtTab = TRUE
andwriteTo
is specified.
Value
A list
containing up to two components:
Indexes
: Atibble::tibble()
ifgtTab = FALSE
(default), orgt::gt()
object ifgtTab = TRUE
, with the desired index(es) for each fitted model (requested via theindexes
argument).GenTEFI
: Atibble::tibble()
of the GenTEFI (if "GenTEFI" is included in theindexes
argument).
Details
If "chisq" is included in
indexes
, the specific form of the applied chi-squared difference test (e.g., standard vs. robust) is determined automatically bylavaan::lavTestLRT()
, based on the model estimation method used.If
gtTab = TRUE
andwriteTo
is specified, then output will simultaneously be saved as a.rtf
file to the user's specified directory.If output file is successfully saved, a confirmation message will be printed to the console.
If a file with the same name already exists in the user's chosen directory, it will be overwritten.
Examples
dvn <- scrapeVarCross(
commitmentQ,
x_order = "spi",
x_stem = "sat.g",
x_delim1 = ".",
x_delim2="_",
distinguish_1="1",
distinguish_2="2"
)
outputUniConstructComp(
dvn,
commitmentQ,
missing = "fiml"
)
#> According to GenTEFI (-15.173),
#> higher-order models (e.g., bifactor or hierarchical) fit the data better than lower-order models (e.g., correlated factors),
#> as the higher-order TEFI (-9.419) is smaller than the lower-order TEFI (-5.754).
#> $Indexes
#> # A tibble: 4 × 14
#> Model Type genTEFI df chisq cfi rmsea bic df_diff chisq_diff
#> <chr> <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 Bifactor High… Yes 20 41.3 0.983 0.0951 4077. NA NA
#> 2 Hierarchical High… Yes 29 49.1 0.984 0.0767 4042. 9 7.78
#> 3 Correlated Fa… Lowe… No 29 49.1 0.984 0.0767 4042. NA NA
#> 4 Unidimensional Lowe… No 30 235. 0.839 0.240 4223. 1 186.
#> # ℹ 4 more variables: chisq_diff_test_p <dbl>, cfi_diff <dbl>,
#> # rmsea_diff <dbl>, bic_diff <dbl>
#>
#> $`GenTEFI Details`
#> # A tibble: 1 × 3
#> VN.Entropy.Fit Lower.Order.VN Higher.Order.VN
#> <dbl> <dbl> <dbl>
#> 1 -15.2 -5.75 -9.42
#>
outputUniConstructComp(
dvn,
commitmentQ,
indexes = c("df", "bic"),
missing = "fiml"
)
#> # A tibble: 4 × 6
#> Model Type df bic df_diff bic_diff
#> <chr> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 Bifactor Higher Order 20 4077. NA NA
#> 2 Hierarchical Higher Order 29 4042. 9 -35.2
#> 3 Correlated Factors Lower Order 29 4042. NA NA
#> 4 Unidimensional Lower Order 30 4223. 1 181.
outputUniConstructComp(
dvn,
commitmentQ,
indexes = c("df", "bic"),
estimator = "ml",
missing = "fiml"
)
#> # A tibble: 4 × 6
#> Model Type df bic df_diff bic_diff
#> <chr> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 Bifactor Higher Order 20 4077. NA NA
#> 2 Hierarchical Higher Order 29 4042. 9 -35.2
#> 3 Correlated Factors Lower Order 29 4042. NA NA
#> 4 Unidimensional Lower Order 30 4223. 1 181.
outputUniConstructComp(
dvn,
commitmentQ,
indexes = c("df", "bic"),
missing = "fiml",
gtTab = TRUE,
writeTo = tempdir(),
fileName = "uni-construct-dyad-models"
)
#> Output stored in: /tmp/RtmpmQWsXV/uni-construct-dyad-models.rtf
Model
Type
df
bic
df_diff
bic_diff